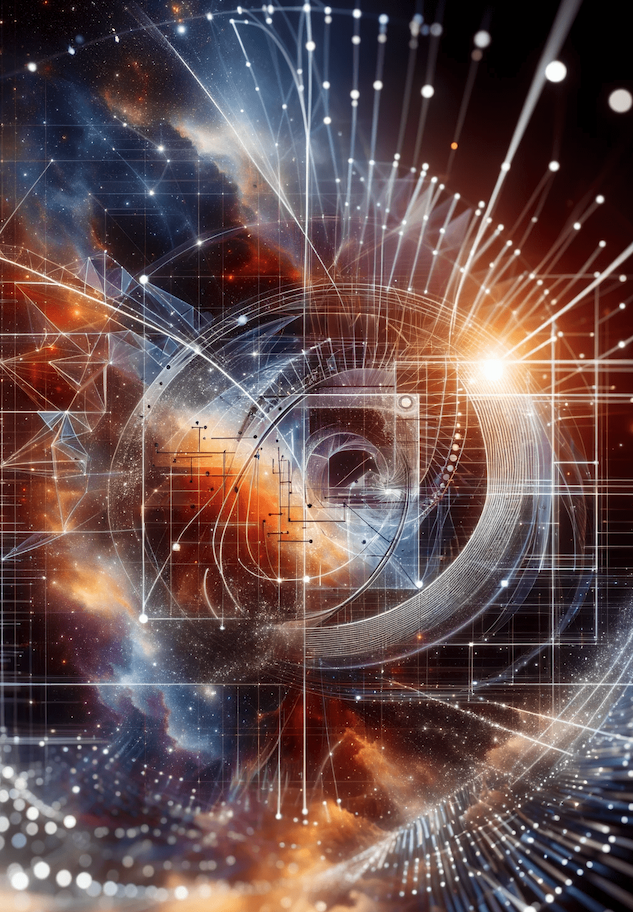
Understanding the distinction between vector...
December 11, 2023
Support center +1-720-229-5567
Object detection is a popular computer vision task that involves detecting and localizing objects within an image or video. In this blog post, we’ll explore how to use transfer learning to train a custom object detection model using TensorFlow, step-by-step, and provide detailed code examples and best practices.
The first step in training a custom object detection model using TensorFlow is to set up the development environment. This involves installing the necessary software and configuring the system to build and train the model.
Here’s an example of the basic setup process:
Install TensorFlow: TensorFlow is an open-source machine learning library developed by Google. You can install TensorFlow using the Python package manager pip, by running the following command in your terminal: pip install tensorflow.
Install the TensorFlow Object Detection API: The TensorFlow Object Detection API is a set of pre-built models and tools that can be used to train custom object detection models. You can install it by following the instructions in the official documentation.
Prepare the Training Data: The training data is a collection of labeled images that the model will use to learn how to detect the target objects. The data should be organized into two directories, one for images and one for labels, and the labels should be in the format expected by the TensorFlow Object Detection API.
Once you have the environment set up and the training data prepared, it’s time to define the model architecture. This involves choosing a pre-trained model as the base and adding new layers to detect the target objects.
Here’s an example of defining the model architecture:
Choose a Pre-Trained Model: The pre-trained model serves as the base for the custom model and provides the initial weights and architecture. You can choose a pre-trained model from the TensorFlow Object Detection API, depending on the task and the complexity of the target objects.
Add New Layers: Once you have the pre-trained model, you can add new layers to the top of the model to detect the target objects. The new layers should be defined using TensorFlow’s high-level Keras API, and they should be trained with the training data.
Here’s an example of adding new layers to a pre-trained model:
import tensorflow as tf
from object_detection.utils import config_util
from object_detection.builders import model_builder
# Load the pre-trained model
pipeline_config = 'path/to/pipeline.config'
config = config_util.get_configs_from_pipeline_file(pipeline_config)
model_config = config['model']
detection_model = model_builder.build(model_config=model_config, is_training=True)
# Add new layers to detect the target objects
num_classes = 2
faster_rcnn = detection_model.faster_rcnn
faster_rcnn.num_classes = num_classes
faster_rcnn.image_resizer_fn = functools.partial(
image_resizer_builder.build, keep_aspect_ratio_resizer_config)
faster_rcnn.feature_extractor = feature_extractor
faster_rcnn.number_of_stages = 2
faster_rcnn.first_stage_anchor_generator.grid_anchor_generator = grid_anchor_generator
faster_rcnn.first_stage_box_predictor_box_coder = box_coder
faster_rcnn.initial_crop_size = init_crop_size
faster_rcnn.maxpool_kernel_size = 2
faster_rcnn.maxpool_stride = 2
faster_rcnn.second_stage_box_predictor = second_stage_box_predictor
faster_rcnn.second_stage_post_processing = second_stage_post_processing
Once you have the model architecture defined, it’s time to train the model using the training data and the newly added layers. This involves using TensorFlow’s built-in training functions and the training data to update the weights of the model.
Here’s an example of training the model:
Load the Training Data: The training data should be loaded into TensorFlow’s dataset API, which provides efficient data loading and preprocessing capabilities.
import tensorflow as tf
image_dir = 'path/to/image/dir'
label_dir = 'path/to/label/dir'
batch_size = 8
image_files = sorted(os.listdir(image_dir))
label_files = sorted(os.listdir(label_dir))
image_paths = [os.path.join(image_dir, file) for file in image_files]
label_paths = [os.path.join(label_dir, file) for file in label_files]
dataset = tf.data.Dataset.from_tensor_slices((image_paths, label_paths))
dataset = dataset.shuffle(buffer_size=len(image_paths))
dataset = dataset.map(parse_fn, num_parallel_calls=tf.data.AUTOTUNE)
dataset = dataset.batch(batch_size)
dataset = dataset.prefetch(buffer_size=tf.data.AUTOTUNE)
Define the Training Loop: The training loop is responsible for updating the weights of the model using the training data. It should use the optimizer to minimize the loss function and evaluate the performance of the model on a validation set.
import tensorflow as tf
num_epochs = 10
learning_rate = 0.001
optimizer = tf.keras.optimizers.Adam(learning_rate=learning_rate)
loss_fn = tf.keras.losses.BinaryCrossentropy()
for epoch in range(num_epochs):
for batch_images, batch_labels in dataset:
with tf.GradientTape() as tape:
predictions = detection_model(batch_images, training=True)
loss = loss_fn(batch_labels, predictions['detection_scores'])
gradients = tape.gradient(loss, detection_model.trainable_variables)
optimizer.apply_gradients(zip(gradients, detection_model.trainable_variables))
Using the Trained Model
Once the model is trained and evaluated, you can use it to detect objects in new images or videos. This involves using the inference function of the model and the test data.
Here’s an example of using the trained model:
import tensorflow as tf
test_image_dir = 'path/to/test/image/dir'
test_batch_size = 8
test_image_files = sorted(os.listdir(test_image_dir))
test_image_paths = [os.path.join(test_image_dir, file) for file in test_image_files]
test_dataset = tf.data.Dataset.from_tensor_slices(test_image_paths)
test_dataset = test_dataset.map(parse_image_fn, num_parallel_calls=tf.data.AUTOTUNE)
test_dataset = test_dataset.batch(test_batch_size)
for images in test_dataset:
detections = detection_model(images, training=False)
# Post-process the detections
Conclusion
In this blog post,we explored how to use transfer learning to train a custom object detection model using TensorFlow, step-by-step. We began by setting up the TensorFlow environment, preparing the training data, defining the model architecture, training the model, and evaluating its performance. Finally, we used the trained model to detect objects in new images or videos.
While there are several libraries and frameworks available for object detection, transfer learning offers a powerful and efficient way to train custom models for specific use cases. By leveraging pre-trained models and adding new layers, you can build highly accurate object detection models with relatively little training data.
In addition, TensorFlow’s built-in training and evaluation functions and its dataset API make it easy to train and test your model on large datasets, and the use of GitHub Actions can make the building and deployment of such models more seamless.
We hope this blog post has provided you with a comprehensive guide to building a custom object detection model using transfer learning and TensorFlow.